이 포스팅은 한국기술교육대학교 김덕수 교수님의 시스템 프로그래밍 (CSE 232)를 참고하여 작성되었습니다.
#1 Low-level vs High-level file IO
Low-Level File IO (System call)
- system call을 이용해서 파일 입출력 수행
- File descriptor 사용
- Byte 단위로 디스크에 입출력
- 특수 파일에 대한 입출력 가능
High-Level File IO (Buffered IO)
- C Standard library를 사용하여 파일 입출력 수행
- File pointer 사용 (FILE *fp)
- 버퍼(block) 단위로 디스크에 입출력 : 여러 형식의 입출력 지원
#2 Opening files - open(2)
man -s 2 open > 인자 1은 shell에 대한 것, 2는 systeam call에 대한 것이다.
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int open(const char *pathname, int flags);
int open(const char *pathname, int flags, mode_t mode);
pathname (file path) : 열려는 파일의 경로 (파일 이름 포함)
flags (file state flags) : 파일 여는 방법(access mode) 설정
- 여러 플래그 조합 가능 (OR bit operation | 사용 )
ex) O_WRONLY | O_TRUNC , O_RDWR | O_APPEND
*man page 참고
mode (file access permission) : 파일을 새로 생성할 때만 유효
- 파일 권한 설정 값 사용 or 정의된 플래그 사용
ex) S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH
*man page 참고
Return : file desctiptor
File descriptor
열려 있는 파일을 구분하는 정수(integer) 값
- 특수 파일 등 대부분의 파일을 지칭 가능
- Process별로 kernel이 관리
파일을 열 때 순차적으로 할당됨
- Process 당 최대 fd 수 = 1,024 (default, 변경 가능)
Default fds (수정 가능)
- 0 : stdin
- 1 : stdout
- 2 : stderr
default fds는 프로세스가 생성되면 기본적으로 할당된다. 🤔
👉 순차적으로 3번부터 fd가 할당된다
File table
열린 파일을 관리하는 표
- Kernel이 process별로 유지
- 열린 파일에 대한 각종 정보 관리
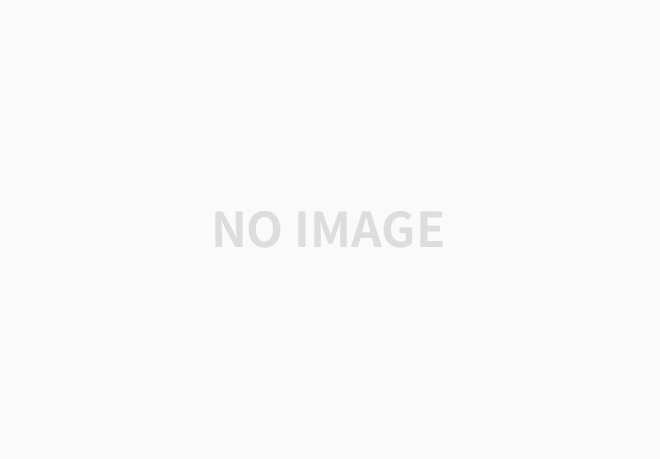
#2 Closing files - close(2)
#include <unistd.h>
int close(int fd);
fd (file descriptor) : 해당 file descriptor의 연결을 끊는다.
Return :
0 = success
-1 = error
Open & Close a file 예제
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdlib.h>
#include <stdio.h>
int main(void) {
int fd;
mode_t mode;
mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH; // 644
fd = open("hello.txt", O_CREAT, mode);
if (fd == -1) {
perror("Creat"); exit(1);
}
close(fd);
return 0;
}
#3 Error handling for system call
- Systeam call은 실패 시 -1을 반환
- Error code는 errno에 저장됨
(1) error.h에 선언되어 있음 (special variable)
(2) extern으로 직접 접근 가능 : extern int errno;
- perror(3) (3은 standard library를 의미한다.)
#include <stdio.h>
void perror(const char *string);
int main(void) {
int fd;
fd= open("hello.txt", O_CREAT | O_EXCL, 0644);
if(fd== -1) {
perror("EXCL");
exit(1);
}
close(fd);
return 0;
}
긴 글 읽어주셔서 감사드립니다.
22.12.13
'TIL (Today I Learned) > 컴퓨터 시스템(CS)' 카테고리의 다른 글
[CS] File, File descriptor #4 (0) | 2022.12.13 |
---|---|
[CS] File, 파일 읽기/쓰기 #3 (0) | 2022.12.13 |
[CS] File, 파일이 무엇인가? #1 (0) | 2022.12.13 |
[CS] 네트워크 기초, HTTP 2 #8 (0) | 2022.12.11 |
[CS] 네트워크 기초, HTTP #7 (0) | 2022.12.11 |
댓글